C++ is a powerful programming language that provides a lot of features to help developers write efficient and effective code. One of these features is pointers. Pointers are a fundamental concept in C++ and play a significant role in the language. They provide a way to manipulate memory directly, which can be a powerful tool for solving complex problems. In this blog post, we will explore the power of pointers in C++ and how they can be used to improve your code.
What are Pointers?
Pointers are variables that store memory addresses. They provide a direct way to access and manipulate memory. A pointer can point to a value stored in memory, allowing you to access and modify it directly. r:
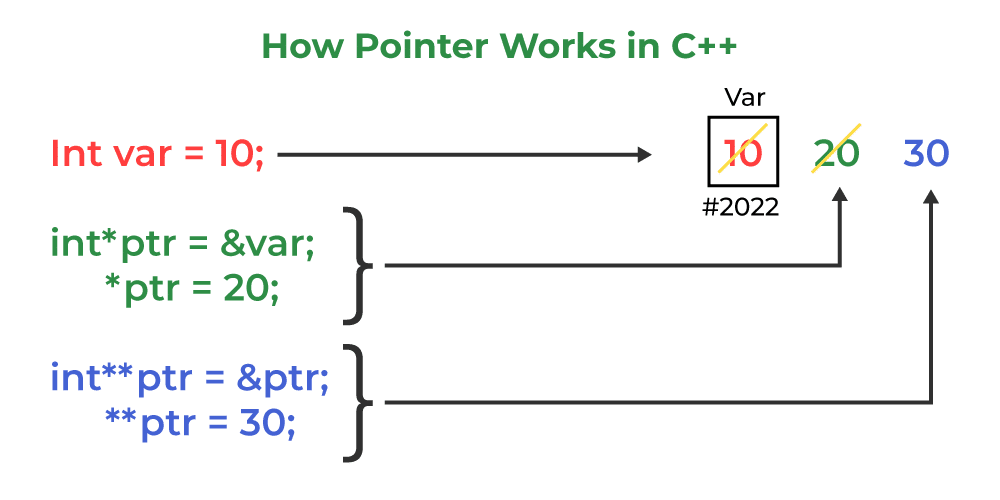
In C++, a pointer is declared by adding an asterisk (*) to the data type of the value it will point to. For example, the following code declares a pointer to an intege
int *ptr;
This declares a pointer named “ptr” that will store the address of an integer value. To assign a value to this pointer, you can use the address-of operator (&) to get the address of a variable, and then assign it to the pointer. For example:
int x = 10;
ptr = &x;
Now, the pointer “ptr” points to the memory location of “x”, and you can access its value using the dereference operator (*). For example:
cout << *ptr << endl; // Outputs 10
Dynamic Memory Allocation
One of the most significant powers of pointers in C++ is their ability to dynamically allocate memory. This means that you can allocate memory during runtime, and it is not limited by the size of the stack or the heap. This feature is particularly useful when you need to allocate a large amount of memory or when you don’t know the size of the data you need to store until runtime.
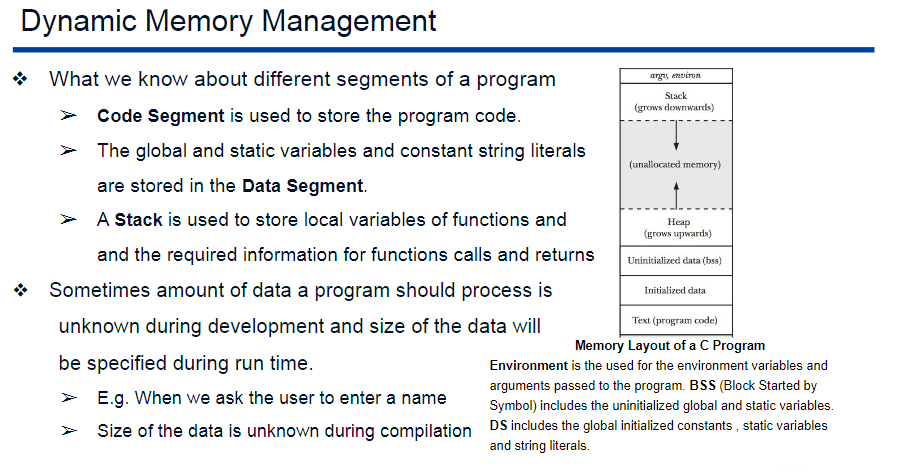
Dynamic memory allocation is done using the “new” operator in C++. For example:
int *ptr = new int;
This will allocate a new integer value in memory and store its address in the pointer “ptr”. You can also allocate arrays of values in a similar way:
int *ptr = new int[10];
This will allocate an array of ten integers and store its address in the pointer “ptr”.
Dynamic memory allocation is particularly useful when you need to allocate a large amount of memory or when you don’t know the size of the data you need to store until runtime. This feature allows you to write more flexible and scalable code, making it easier to handle complex problems.
Functions and Pointers
Pointers can also be used to pass information to functions in C++. You can pass a pointer as an argument to a function and use it to access and modify values stored in memory. This provides a way to pass information between functions and allows you to write more flexible and reusable code.
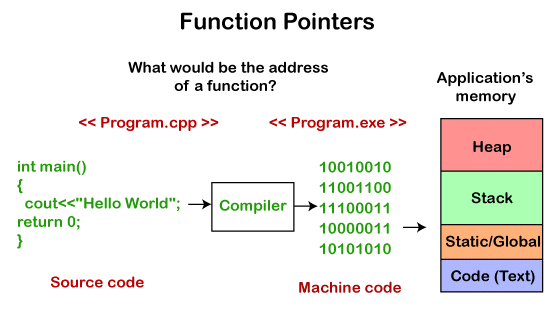
For example, consider the following code:
void swap(int *a, int *b)
{
int temp = *a;
*a = *b;
*b = temp;
}
This function takes two pointers to integers as arguments and swaps their values. You can call this function in the following way:
int x = 10, y = 20;
swap(&
Summary
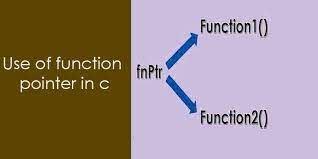
Pointers are a fundamental concept in C++ that allow for direct manipulation of memory. They are declared by adding an asterisk (*) to the data type of the value it will point to. Pointers can be used to dynamically allocate memory using the “new” operator, improving the flexibility and scalability of code. They can also be passed as arguments to functions to pass information between them, making code more flexible and reusable. Understanding and utilizing pointers is a key aspect of writing effective code in C++.